Mastering Selenium with Python: A Comprehensive Guide
Written on
Chapter 1: Introduction to Selenium
Selenium is a powerful suite designed for automating web browsers. It serves a variety of purposes, including testing applications, browsing the web, performing actions online, and scraping data from websites. This guide provides a concise tutorial demonstrating basic applications of Selenium using Python, specifically tailored for automatic navigation to Instagram.com.
Installing Selenium
To begin, installing Selenium is straightforward via pip. Additionally, you'll require a Chrome WebDriver that matches your version of Chrome. You can check your version by visiting chrome://settings/help. Alternatively, you can utilize the webdriver-manager module, which can also be installed with pip:
$ pip install selenium
$ pip install webdriver_manager
Creating Your First Web Bot
Start by creating a Python file, which I named "instagram.py." Import the Selenium WebDriver and ChromeDriverManager into your project as shown below:
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
Initiating the Browser
To launch the browser, use the following command:
browser = webdriver.Chrome(ChromeDriverManager().install())
Opening a Website
You can navigate to any website using the command browser.get('URL'). For instance, to access Instagram's sign-in page, use the following code:
Once you run the script, a Chrome window will open, displaying the Instagram sign-in page.
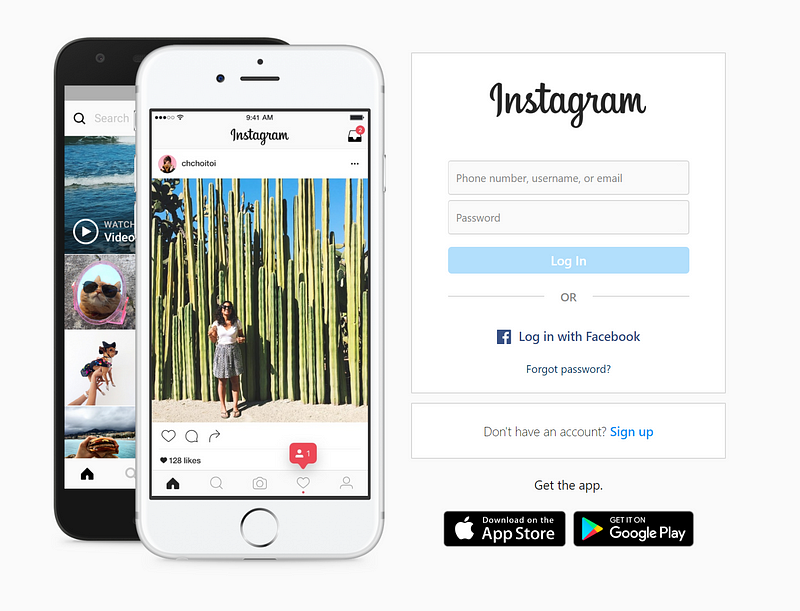
Locating Web Elements
Next, program the Selenium browser to find specific elements and interact with them. You can locate elements using various methods:
- find_element_by_id
- find_element_by_name
- find_element_by_xpath
- find_element_by_link_text
- find_element_by_partial_link_text
- find_element_by_tag_name
- find_element_by_class_name
- find_element_by_css_selector
In this tutorial, we will explore Instagram.com. After the login page appears, you can identify the class of the button by opening Developer Mode (press F12).
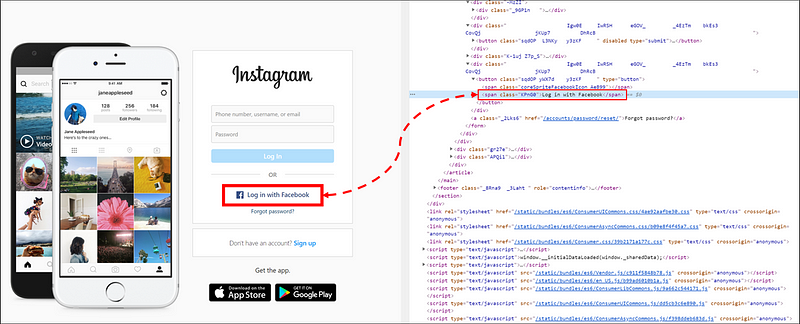
The "Sign in with Facebook" button has the class name KPnG0. We can instruct the browser to find this element by its class name and click on it:
browser.find_element_by_class_name('KPnG0').click()
When you execute the script at this point, the Facebook login page will appear in your Selenium browser.
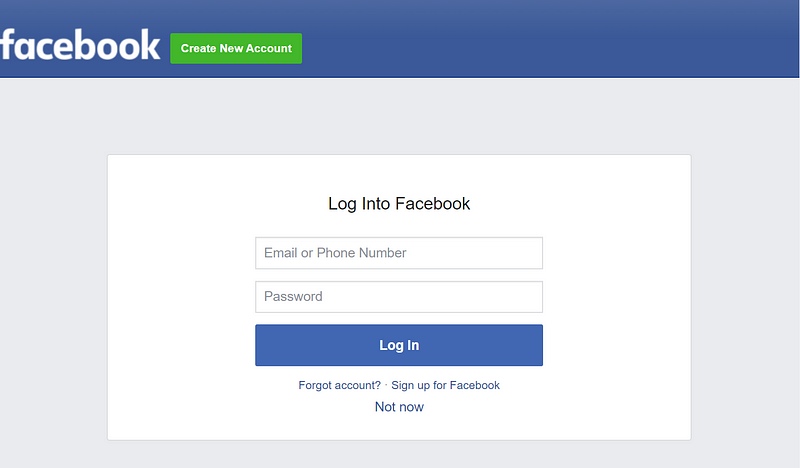
Sending Keys
Selenium allows you to send key inputs to specific elements on the browser using the command element.send_keys("..."). In this case, we will input the "Email or Phone number" and "Password" fields and then click the "Log In" button. We'll locate these elements using their names.
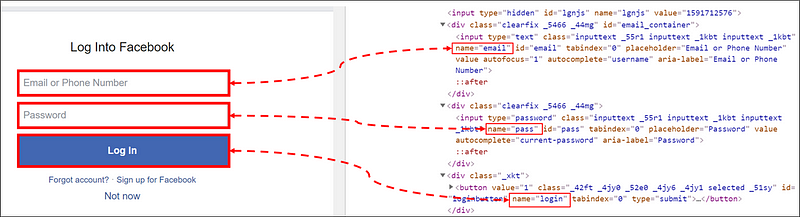
fb_name = "Your Facebook Email (or phone)"
fb_pass = "Your Facebook password"
# Fill credentials
browser.find_element_by_name("email").send_keys(fb_name)
browser.find_element_by_name("pass").send_keys(fb_pass)
# Click Log In
browser.find_element_by_id('loginbutton').click()
After running the script, you should successfully log in to Instagram using your Facebook credentials. A "Turn on Notifications" prompt may appear; you can dismiss it by clicking the "Not Now" button, which you can locate using its XPath in Developer Mode.
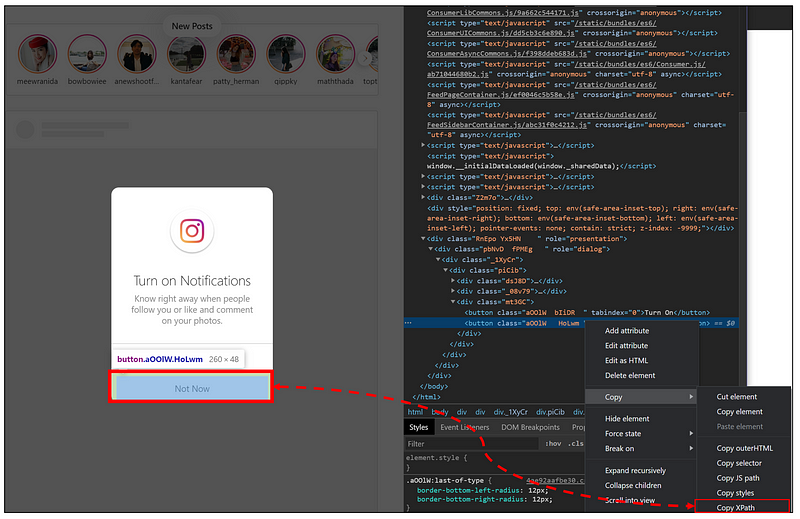
# Click on "Not Now"
browser.find_element_by_xpath('/html/body/div[4]/div/div/div/div[3]/button[2]').click()
Conclusion
Congratulations! Your Selenium browser is now logged into your Instagram account using your Facebook credentials. You can also adapt this method to log in with your email and password or use it on other websites.
Combining everything we've covered, the overall script appears as follows. With this foundation, you can explore further functionalities like retrieving follower data, following back users, and much more.
See it in action:
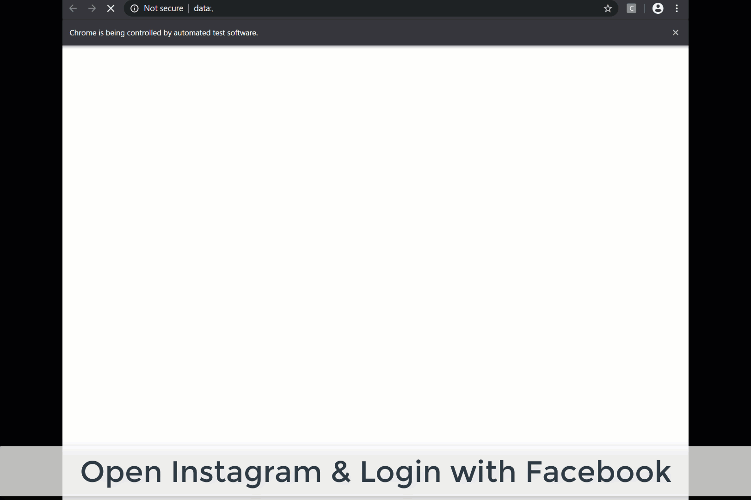
For a visual demonstration, check out the video titled "Python Selenium Full Series - Getting Started [Web Bots and Testing]" on YouTube.
Additionally, watch "Python Selenium Tutorial - Automate Websites and Create Bots" for more insights into using Selenium effectively.
Thank you for reading! Feel free to reach out if you have any questions or need assistance.