Understanding Python's Custom Slicing with a Dog Class
Written on
Chapter 1: Introduction to Custom Slicing
In Python, you may have encountered slicing in libraries like pandas, which allows for selecting specific rows and columns. For instance:
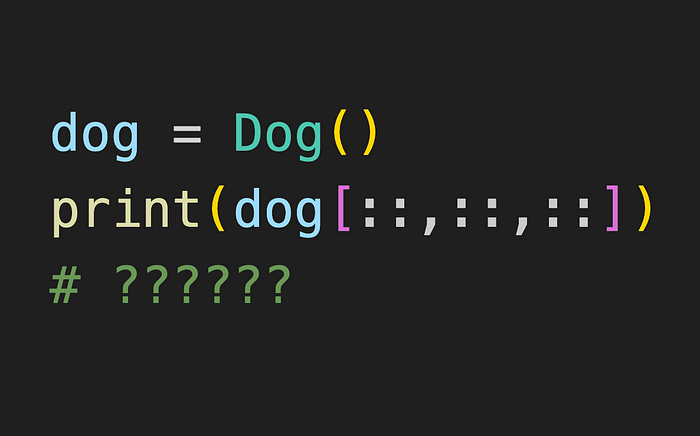
# df represents a pandas DataFrame
df.iloc[:, :3]
This syntax enables the selection of particular rows and columns. However, if we attempt to use a custom class like Dog, we have to define how it handles slicing.
Section 1.1: Default Behavior of Dog Class
class Dog:
pass
dog = Dog()
print(dog[::, ::, ::]) # This results in an error
By default, trying to slice the Dog instance leads to an error, as the class lacks any slicing implementation.
Subsection 1.1.1: Implementing __getitem__
To enable slicing behavior, we define the __getitem__ method:
class Dog:
def __getitem__(self, key):
return key
dog = Dog()
print(dog[1]) # Outputs: 1
print(dog[2]) # Outputs: 2
print(dog['apple']) # Outputs: apple
This method allows the Dog object to return the key itself, meaning that calling dog[1] gives back 1, and dog['apple'] returns 'apple'.
Section 1.2: Understanding Slicing with Dog
When we execute:
print(dog[::]) # Outputs: slice(None, None, None)
This indicates that Python is passing a slice object to the __getitem__ method, akin to how it handles lists or strings.
Chapter 2: Advanced Slicing Techniques
Let's explore how to manage multiple arguments in our custom class.
class Dog:
def __getitem__(self, key):
return key
dog = Dog()
print(dog[1]) # Outputs: (1,)
print(dog[1, 2]) # Outputs: (1, 2)
print(dog[1, 2, 3, 4, 5]) # Outputs: (1, 2, 3, 4, 5)
If we call dog[1, 2, 3], the tuple (1, 2, 3) is sent to the __getitem__ method.
The first video titled "Florida Python Dog Finds A Python Nest Infested With Giant Ticks" showcases a dog discovering a python nest filled with ticks, illustrating the fascinating interactions between wildlife and their environments.
In the second video, "Florida Python Dog Finds Huge Python Laying On Underground Nest," we see how a dog uncovers a significant python resting on its nest, highlighting the unique relationship between dogs and pythons in Florida.
Conclusion
By customizing your classes and defining how they handle slicing, you can create more complex and intuitive behaviors. This example of the Dog class demonstrates the flexibility of Python's slicing capabilities.
If you enjoyed this content, consider supporting me as a creator! A simple clap or a comment about your favorite part makes a big difference. Thank you for your support!