Build Your Own SMS Alert System Using Spring Boot and Twilio
Written on
Introduction to SMS Alert Systems
Creating a personalized alert system is surprisingly simple and offers endless possibilities. In today’s digital landscape, we receive notifications from various platforms like WhatsApp, Instagram, and Snapchat. These alerts keep us informed about significant events through in-app notifications, emails, and SMS.
Have you ever considered developing your own alert system? In this guide, I will demonstrate how to construct an SMS alert system that activates whenever an API is triggered.
To achieve this, we will utilize Twilio, a customer engagement platform that many developers use to enhance user experiences.
Prerequisites for Getting Started
Before diving into this project, it’s essential to have a foundational understanding of Java, Maven, and Spring Boot.
The tools and versions I will use for this project include:
- Java 11
- Spring Boot 2.7.x
- Apache Maven 3.6.3
- Twilio account
- IntelliJ IDEA Community Edition (IDE)
Creating a Free Twilio Account
To begin, you'll need to sign up for a free account with Twilio, where you will set up your phone number in the following steps. No credit card is necessary for creating the free account.
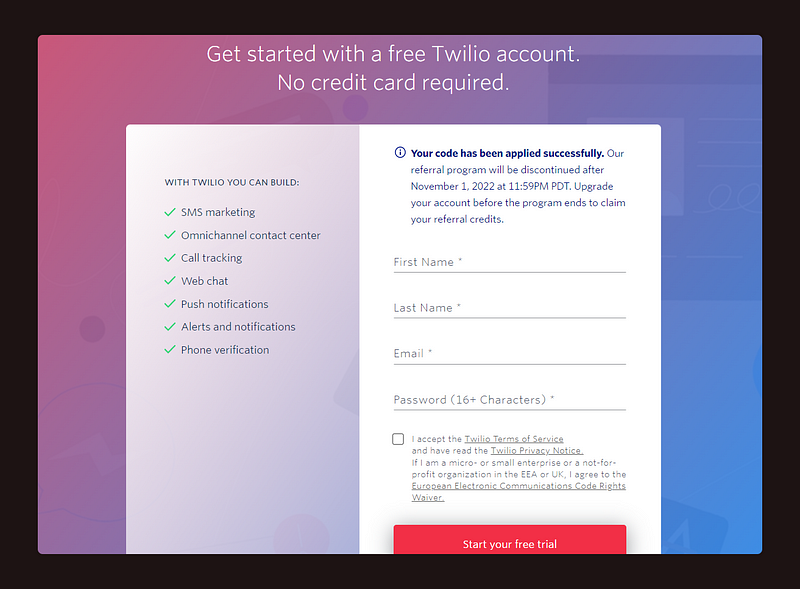
After filling in your details and verifying your email, you'll need to add and confirm your phone number. This is where your SMS alert system will be activated.
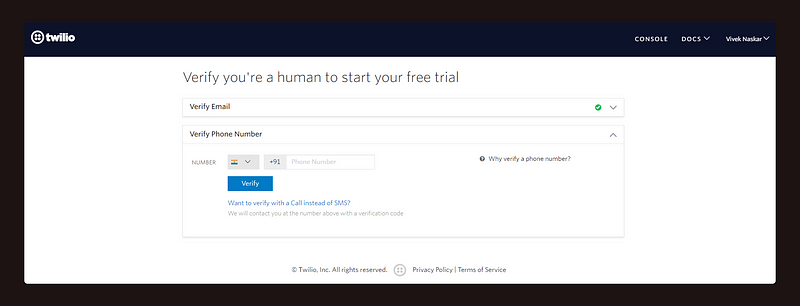
Once your phone number is verified, you should see a welcome page. Complete the required details on the form before clicking the "Get Started with Twilio" button.
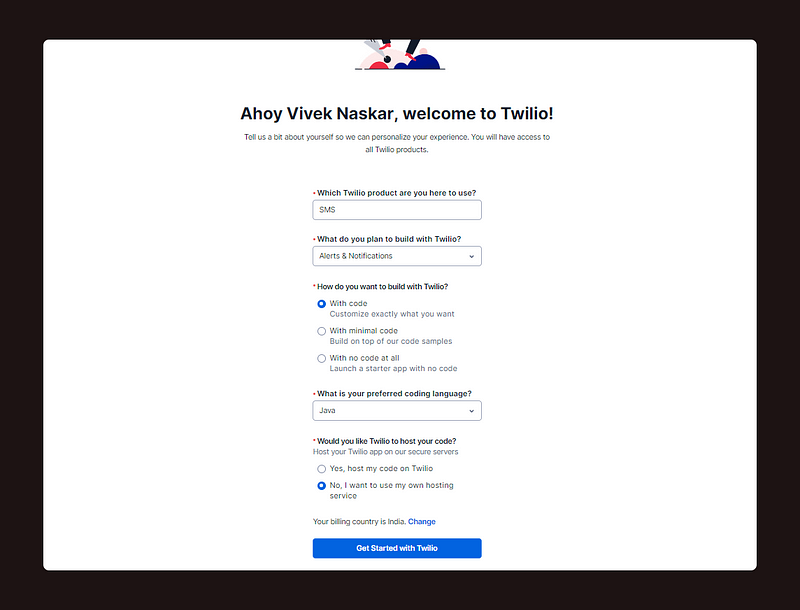
The Twilio Console will appear as shown below:
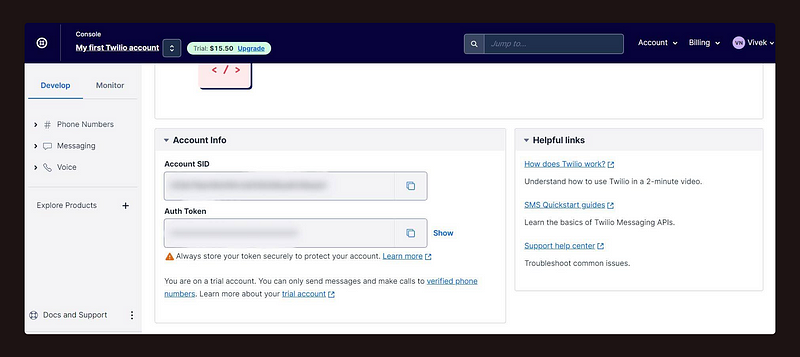
Keep your Account SID and Auth Token confidential, as these credentials grant access to your Twilio account. We will use these fields in the upcoming steps. In the console, you’ll find a button labeled “Get a trial phone number,” which allows you to obtain a valid Twilio phone number needed for sending SMS messages.
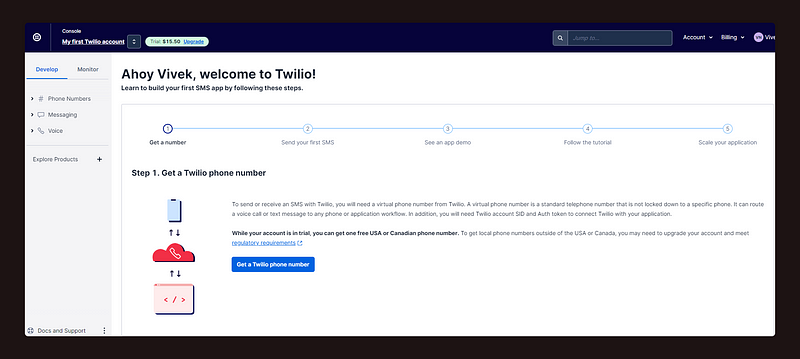
Once you generate your trial phone number, it will be displayed in the Twilio console alongside your Account SID and Auth Token.
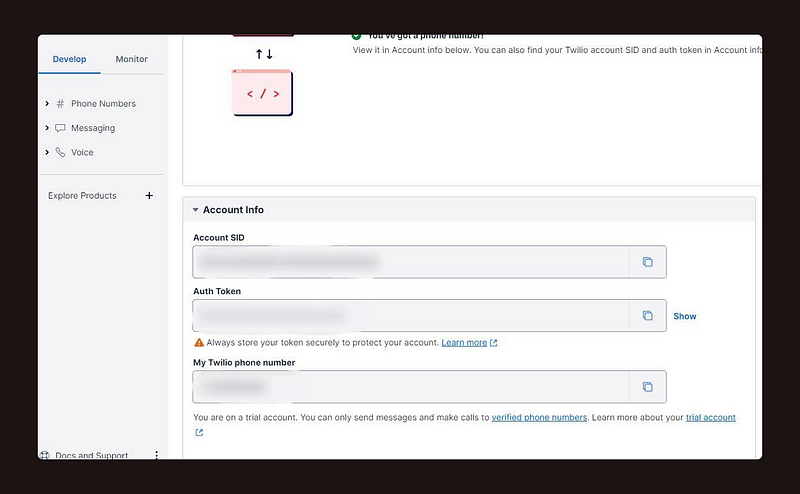
Setting Up Your Spring Boot Application
To start, download a blank demo application from Spring Initializer. Next, add the Twilio SDK dependency to your Spring Boot project's pom.xml file:
<dependency>
<groupId>com.twilio.sdk</groupId>
<artifactId>twilio</artifactId>
<version>9.0.1</version> <!-- Ensure to use the latest version -->
</dependency>
Consequently, your complete dependencies list in pom.xml will look like this:
Building the SMS Alert System
For this simple application, I will use a single controller that accepts the alert message as the payload, allowing for customization.
To use the Twilio SDK, initialize your credentials with the init() method annotated with @PostConstruct.
The Account SID and Auth Token will be retrieved using the @Value annotation from the application.properties file.
The core functionality for sending SMS is implemented using the static method Message.creator(param1, param2, param3), where:
- param1 is the recipient's phone number,
- param2 is the sender's Twilio phone number,
- param3 is the message content.
This functionality is encapsulated in the sendSMS() method of the AlertServiceImpl class, which implements the AlertService interface.
As previously mentioned, the controller class straightforwardly invokes the sendSMS() method from the AlertService.
Testing the SMS Alert System
Once the application is up and running, you can use Postman to test it with your custom message.
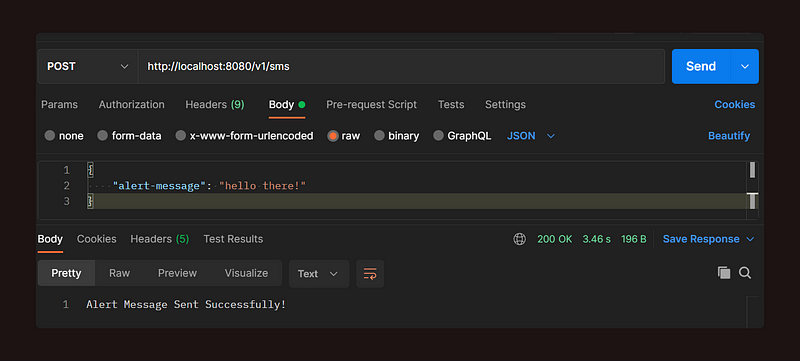
If successful, you will receive the SMS on your phone.
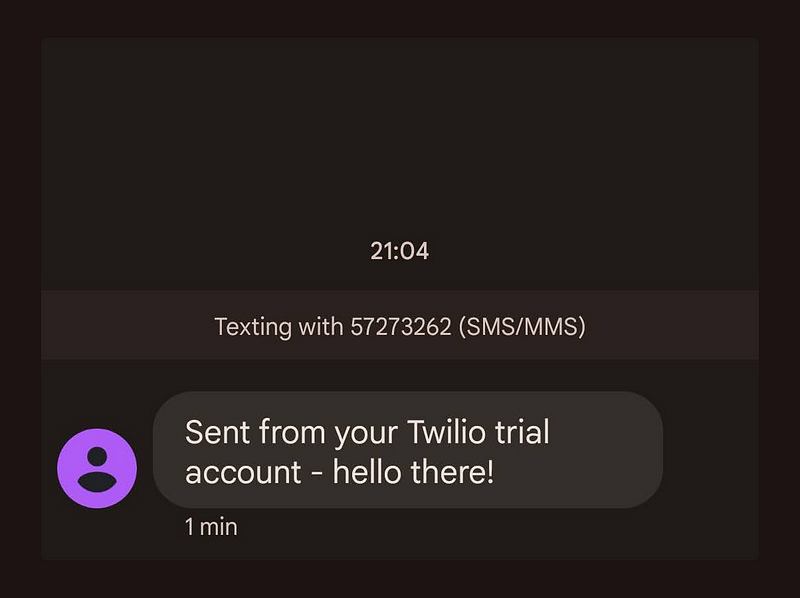
Congratulations! You’ve successfully sent your first SMS using Spring Boot.
I thoroughly enjoyed working on this article, and I hope you find joy in creating your own messaging system. This guide merely scratches the surface—there's so much more to explore. Feel free to experiment with the code, such as sending messages to multiple recipients or extracting the recipient's phone number from the payload.
If you appreciated this content, you might also find the following articles interesting:
Everything You Need To Know About The CompletableFuture API
The API that made asynchronous programming exciting in Java!
levelup.gitconnected.com
5 Important Lessons That Every Software Developer Should Know & Learn
Becoming a better software developer is a journey worth taking.
levelup.gitconnected.com
If you enjoy learning through stories that enhance your work and life, consider subscribing. If this article was helpful, please support my work—only if you are able. You can also connect with me on X. Thank you!
Chapter 2: Video Tutorials on SMS Alert Systems
In this video, you will learn how to effortlessly send SMS messages using Spring Boot and Twilio.
This tutorial provides a comprehensive guide on sending SMS using the Twilio API with Spring Boot.