Mastering Regular Expressions in JavaScript for Substring Extraction
Written on
Chapter 1: Introduction to Regular Expressions
In the world of JavaScript, there are times when we need to extract specific substrings found within parentheses. This guide will explore how to leverage regular expressions to achieve this task efficiently.
This paragraph will result in an indented block of text, typically used for quoting other text.
Section 1.1: The Basics of Regular Expressions
Regular expressions (regex) are powerful tools that allow developers to search and manipulate strings based on specific patterns. To find substrings nestled between parentheses, we can utilize the match method combined with a well-structured regex pattern.
For example:
const txt = "I expect five hundred dollars ($500) and new brackets ($600)";
const regExp = /(([^)]+))/g;
const matches = [...txt.match(regExp)];
console.log(matches);
In this snippet, the regex pattern /(([^)]+))/g is designed to capture all characters located between parentheses.
Subsection 1.1.1: Understanding the Regex Pattern
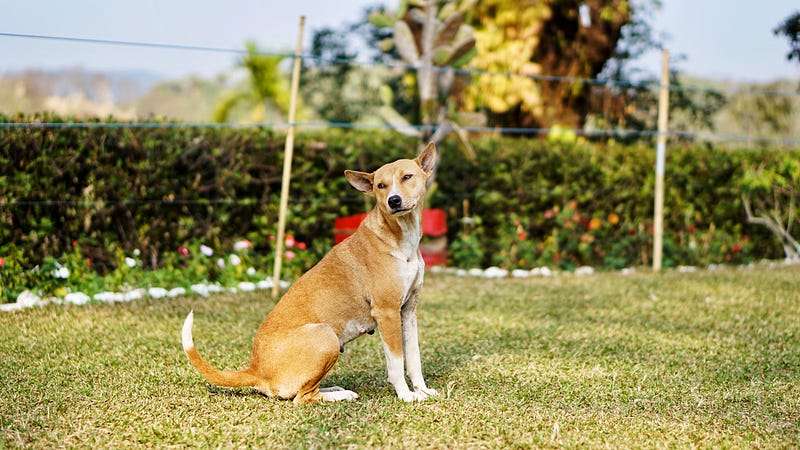
The g flag signifies that the search will encompass the entire string, identifying every instance that meets the criteria. As a result, matches will yield an array containing ["($500)", "($600)"].
Section 1.2: Excluding Inner Parentheses
To refine our search and avoid capturing substrings with inner parentheses, we can modify our regex to: /(([^()]*))/g.
Chapter 2: Advanced Techniques in Regex
For a more refined extraction of substrings devoid of parentheses, the matchAll method can be employed, as shown below:
const txt = "I expect five hundred dollars ($500) and new brackets ($600)";
const regExp = /(([^)]+))/g;
const matches = [...txt.matchAll(regExp)].flat();
console.log(matches);
This code flattens the resulting array to give us: ["($500)", "$500", "($600)", "$600"].
The first video titled "What do () parenthesis and | pipe mean in RegExp? #2 REGEX ULTRA BASICS" provides an in-depth explanation of regex fundamentals and their significance in programming.
The second video, "Regular Expressions (Regex) Tutorial: How to Match Any Pattern of Text," elaborates on various regex techniques to match text patterns effectively.
Conclusion
In summary, harnessing the power of regular expressions enables JavaScript developers to efficiently extract substrings located within parentheses. This skill is invaluable for various programming tasks.
Further Reading
Best Tool for Web Scraping: BeautifulSoup vs. Regex vs. Advanced Web Scrapers
Explore the strengths of BeautifulSoup, Regular Expressions, and advanced web scraping methods to find the best tool for your needs.
More content at PlainEnglish.io. Sign up for our free weekly newsletter. Follow us on Twitter, LinkedIn, YouTube, and Discord.
Interested in scaling your software startup? Check out Circuit.