The Evolution of JAMstack Architecture in Web Development
Written on
Understanding JAMstack Architecture
In recent years, the web development field has undergone significant changes. Traditional monolithic frameworks are being replaced by more modular and efficient systems. A standout advancement in this evolution is the JAMstack architecture. JAMstack, which stands for JavaScript, APIs, and Markup, provides a contemporary method for creating fast, scalable, and secure websites. This article will delve into what JAMstack is, its advantages, and how to construct a site using this innovative framework.
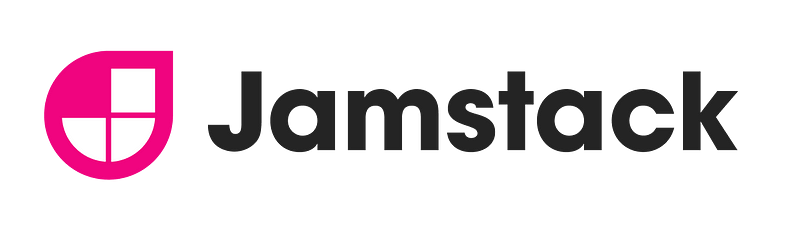
What Constitutes JAMstack?
JAMstack represents a modern architecture for web development that relies on client-side JavaScript, reusable APIs, and pre-rendered Markup. Unlike traditional monolithic designs that tightly integrate the front-end and back-end, JAMstack separates these elements, allowing developers to craft more efficient and scalable websites.
The Shift in Web Development Paradigms
Web development historically relied on server-side rendering, where HTML was generated by the server for each request. This method worked effectively for early web applications but faced scalability and performance challenges as complexity grew. The rise of client-side JavaScript frameworks such as React, Angular, and Vue initiated a major shift towards Single Page Applications (SPAs). While SPAs enhanced user experiences through dynamic content loading, they also introduced new hurdles like intricate state management and prolonged initial load times.
JAMstack emerged to address these issues by merging the benefits of static sites with the interactivity of dynamic applications.
Key Elements of JAMstack
- JavaScript: Manages dynamic functionality on the client side using frameworks like React, Vue, or plain JavaScript.
- APIs: Act as backend services accessed through HTTPS, which can be third-party services or custom-built serverless functions.
- Markup: Refers to pre-rendered HTML generated by static site generators such as Jekyll, Hugo, or Gatsby.
Benefits of Adopting JAMstack
Performance
One of the major advantages of JAMstack is its performance. Since the content is pre-built and delivered via a Content Delivery Network (CDN), users enjoy quicker load times and enhanced overall performance. This approach negates the need for complicated server-side rendering, which reduces latency and improves user experience.
Security
JAMstack websites are naturally more secure as they do not depend on traditional server-side architectures. By serving static files and delegating dynamic functionalities to APIs, the attack surface is significantly minimized, making it more difficult for cybercriminals to exploit vulnerabilities common in conventional web applications.
Scalability
Another significant benefit of JAMstack is its scalability. Static files can be effortlessly distributed across multiple servers via CDNs, allowing the site to manage high traffic volumes without sacrificing performance. Additionally, APIs can be scaled independently, enabling developers to optimize various application components as necessary.
Enhanced Developer Experience
JAMstack also improves the developer experience by fostering a modular and decoupled architecture. Developers can leverage modern tools and workflows, such as Git-based version control and automated builds, to simplify the development process. This model encourages the use of reusable components and APIs, resulting in more maintainable and scalable codebases.
Getting Started with JAMstack
Selecting the Appropriate Tools
Before embarking on building a JAMstack website, it’s vital to select the right tools. Here’s a brief overview of popular tools and technologies within the JAMstack ecosystem:
- Static Site Generators (SSGs): Jekyll, Hugo, Gatsby, Next.js
- JavaScript Frameworks: React, Vue, Angular
- APIs and Headless CMS: Contentful, Sanity, Strapi, Netlify Functions
- Hosting and Deployment: Netlify, Vercel, GitHub Pages
Establishing the Development Environment
To begin, set up your development environment with the necessary tools and dependencies. This typically includes installing Node.js, a package manager like npm or yarn, and your chosen static site generator and JavaScript framework.
Here’s a brief example of setting up a JAMstack project using Gatsby:
- Install Node.js and npm: Download Node.js from the official site and verify the installation by running node -v and npm -v in your terminal.
- Install Gatsby CLI: Run npm install -g gatsby-cli.
- Create a New Gatsby Project: Execute gatsby new my-jamstack-site, then navigate into your project directory with cd my-jamstack-site.
- Start the Development Server: Use gatsby develop to launch the server.
Your Gatsby project is now ready for building your JAMstack website.
An introduction to the JAMstack architecture for building websites. This video provides a foundational overview of JAMstack, discussing its principles and applications.
Building a JAMstack Website
Structuring Your Project
A well-organized project structure is essential for maintaining a scalable and manageable codebase. Below is an example structure for a JAMstack project utilizing Gatsby:
my-jamstack-site/
├── src/
│ ├── components/
│ │ └── Header.js
│ ├── pages/
│ │ ├── index.js
│ │ └── about.js
│ └── styles/
│ └── global.css
├── static/
│ └── images/
├── gatsby-config.js
└── package.json
Creating Markup with Static Site Generators
Static site generators (SSGs) are integral to JAMstack. They allow for the pre-building of HTML pages from templates and content files. Here’s how to create a simple homepage and an about page with Gatsby.
Homepage (src/pages/index.js):
import React from "react";
import Header from "../components/Header";
const IndexPage = () => (
<div>
<Header />
<main>
<h1>Welcome to My JAMstack Site</h1>
<p>This is the homepage.</p>
</main>
</div>
);
export default IndexPage;
About Page (src/pages/about.js):
import React from "react";
import Header from "../components/Header";
const AboutPage = () => (
<div>
<Header />
<main>
<h1>About Us</h1>
<p>This is the about page.</p>
</main>
</div>
);
export default AboutPage;
Header Component (src/components/Header.js):
import React from "react";
import { Link } from "gatsby";
const Header = () => (
<header>
<nav>
<ul>
<li><Link to="/">Home</Link></li>
<li><Link to="/about">About</Link></li>
</ul>
</nav>
</header>
);
export default Header;
Adding Interactivity with JavaScript
Interactivity is vital for modern web applications. With JAMstack, you can utilize client-side JavaScript to introduce dynamic behavior to static pages. For instance, let’s add a simple counter component to our homepage.
Counter Component (src/components/Counter.js):
import React, { useState } from "react";
const Counter = () => {
const [count, setCount] = useState(0);
return (
<div>
<p>Current Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
export default Counter;
Updating the Homepage (src/pages/index.js):
import React from "react";
import Header from "../components/Header";
import Counter from "../components/Counter";
const IndexPage = () => (
<div>
<Header />
<main>
<h1>Welcome to My JAMstack Site</h1>
<p>This is the homepage.</p>
<Counter />
</main>
</div>
);
export default IndexPage;
Integrating APIs for Dynamic Features
APIs are crucial in JAMstack, providing dynamic capabilities to static sites. Let’s demonstrate how to integrate a third-party API to fetch and display data.
We’ll utilize the JSONPlaceholder API to retrieve and display a list of posts.
Fetching and Displaying Data (src/pages/posts.js):
import React, { useEffect, useState } from "react";
import Header from "../components/Header";
const PostsPage = () => {
const [posts, setPosts] = useState([]);
useEffect(() => {
.then(response => response.json())
.then(data => setPosts(data));
}, []);
return (
<div>
<Header />
<main>
<h1>Posts</h1>
<ul>
{posts.map(post => (
<li key={post.id}>
<h2>{post.title}</h2>
<p>{post.body}</p>
</li>
))}
</ul>
</main>
</div>
);
};
export default PostsPage;
Don’t forget to add a link to the Posts page in the Header component.
Deploying Your JAMstack Website
Hosting Choices
One of the benefits of JAMstack is its flexibility in hosting. Since the site is pre-built, it can be hosted on any static file server or CDN. Notable options include:
- Netlify: Offers continuous deployment, serverless functions, and a global CDN.
- Vercel: Renowned for its seamless integration with Next.js and static site hosting.
- GitHub Pages: A free option for hosting static sites directly from a GitHub repository.
Continuous Deployment with Git-Based Workflows
Continuous deployment is an essential feature of JAMstack. By integrating with version control systems like Git, you can automate deployment processes. Here’s how to set up continuous deployment with Netlify:
- Connect Your Repository: On the Netlify dashboard, create a new site and link your GitHub repository.
- Configure Build Settings: Specify the build command and publish directory. For a Gatsby site, the build command is gatsby build, and the publish directory is public.
- Deploy: Netlify will automatically build and deploy your site whenever changes are pushed to the repository.
Simplifying Your Web Architecture With Jamstack. This video outlines how JAMstack can streamline your web architecture for enhanced performance and security.
Advanced JAMstack Concepts
Managing User Authentication
User authentication poses challenges in a JAMstack architecture due to the decoupled backend. However, various services and strategies can help manage authentication effectively:
- Auth0: A popular authentication service that integrates well with JAMstack applications.
- Firebase Authentication: Offers a straightforward and secure method for user management and authentication.
Here’s how to integrate Auth0 in a React-based JAMstack site:
- Install Auth0 SDK: npm install @auth0/auth0-react.
- Configure Auth0:
import React from "react";
import { Auth0Provider } from "@auth0/auth0-react";
const App = ({ children }) => (
<Auth0Provider
domain="YOUR_AUTH0_DOMAIN"
clientId="YOUR_AUTH0_CLIENT_ID"
redirectUri={window.location.origin}
>
{children}</Auth0Provider>
);
export default App;
- Utilize Auth0 Hooks:
import React from "react";
import { useAuth0 } from "@auth0/auth0-react";
const LoginButton = () => {
const { loginWithRedirect } = useAuth0();
return <button onClick={() => loginWithRedirect()}>Log In</button>;
};
export default LoginButton;
Performance Optimization Strategies
Optimizing performance is vital for a successful JAMstack site. Here are several techniques:
- Image Optimization: Use tools like Imgix or Cloudinary to deliver optimized images.
- Code Splitting: Divide your JavaScript bundles to load only what is necessary for each page.
- Lazy Loading: Load non-essential resources lazily to enhance initial load times.
- Prefetching: Prefetch resources and pages that users are likely to access next.
Implementing Serverless Functions
Serverless functions provide a powerful way to add backend capabilities to your JAMstack site without server management. Netlify Functions and AWS Lambda are popular choices. Here’s a simple example of a serverless function using Netlify:
- Create a Function File: Add a file in the functions directory (e.g., hello.js):
exports.handler = async (event, context) => {
return {
statusCode: 200,
body: JSON.stringify({ message: "Hello, world!" }),
};
};
- Deploy and Use the Function: Netlify will automatically deploy the function. You can call it from your frontend:
fetch("/.netlify/functions/hello")
.then(response => response.json())
.then(data => console.log(data.message));
The Future of JAMstack
Emerging Trends and Technologies
The JAMstack ecosystem is continuously evolving, with new tools and technologies regularly emerging. Notable trends to keep an eye on include:
- Edge Computing: Bringing computation closer to users for quicker response times.
- Enhanced Developer Tooling: Improved tools and plugins for a better development experience.
- Integration with Modern Frameworks: Deeper ties with frameworks like Next.js and Nuxt.js.
Predictions for the Future
As web development continues to advance, JAMstack is likely to become the standard for crafting modern websites. Its focus on performance, security, and scalability makes it an appealing choice for developers and businesses alike.
JAMstack signifies a notable shift in website development and deployment. By decoupling front-end and back-end elements, leveraging static site generators, and utilizing APIs, developers can create fast, secure, and scalable web applications. Whether you’re working on a personal blog or a large-scale enterprise solution, JAMstack offers a robust and flexible architecture to suit your needs.
As you begin your journey with JAMstack, take the time to explore the vast array of tools and services available. Experiment with different static site generators, integrate various APIs, and deploy your site using modern hosting solutions. The opportunities are limitless, and the future of web development shines brightly with JAMstack.
For more articles like this, follow me on Medium, or subscribe to receive my latest stories via email. You might also want to explore my lists or check out related articles like:
- Step-by-Step Guide to Developing Multilingual Landing Pages Using React, Vite, and Tailwind CSS
- Getting Started with Vue: A Beginner’s Guide
- React Suspense Explained: Mastering Asynchronous UI Handling in React
- React vs. Vue in 2024: A Detailed Framework Comparison for Web Developers